Unit test are crucial part in software development process. In late 1990s Kent Beck stated that writing tests is the most important part of writing software in ExtremeProgramming
This is a part of a series of articles about writing a perfect console application in .net core 2. Feel free to read more:
My example
To have a simple
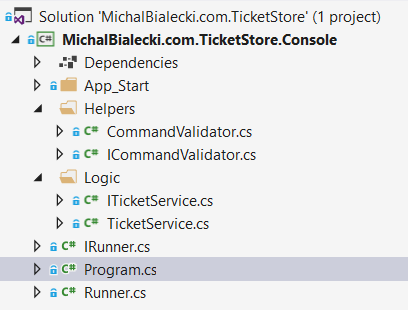
Here is how command handling looks like:
command = Console.ReadLine(); if (!_commandValidator.IsValid(command)) { Console.WriteLine($"Sorry, command: '{command}' not recognized."); }
And CommandValidator looks like this:
As you noticed validator contains regular expression and parsing logic, that can always be faulty. We only allow a column to be from A to H and seat to be between 1 and 15. Let’s add unit test to be sure that it works that way.
Adding tests project
Adding a test project
Project name that I would like to test is MichalBialecki.com.TicketStore.Console so I need to add a class library with
MichalBialecki.com.TicketStore.Console.Tests.
Adding unit tests packages
To write unit tests I’m adding my favourite packages:
- NUnit – unit test framework
- NUnit3TestAdapter – package to run tests
- NSubstitute – mocking framework
- Microsoft.NET.Test.Sdk – it’s important to remember about this one, tests would not run without it
Now we can start writing tests.
First test
I added a CommandValidatorTests and now my project structure looks like this:
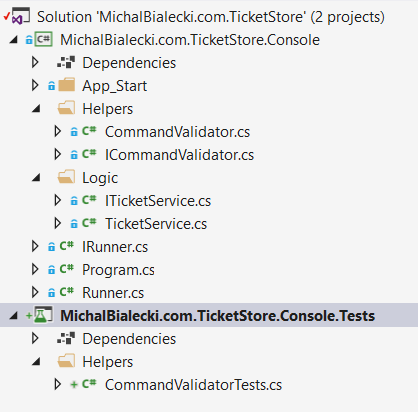
And test looks like this.
using MichalBialecki.com.TicketStore.Console.Helpers; using NUnit.Framework; [TestFixture] public class CommandValidatorTests { private CommandValidator _commandValidator; [SetUp] public void SetUp() { _commandValidator = new CommandValidator(); } [TestCase("A1", true)] [TestCase("A15", true)] [TestCase("A11", true)] [TestCase("H15", true)] [TestCase("H16", false)] [TestCase("K15", false)] [TestCase("I4", false)] [TestCase("K.", false)] [TestCase("", false)] [TestCase(null, false)] public void IsValid_GivenCommand_ReturnsExpectedResult(string command, bool expectedResult) { // Arrange & Act var result = _commandValidator.IsValid(command); // Assert Assert.AreEqual(expectedResult, result); } }
In Resharper unit tests sessions window all tests passed.
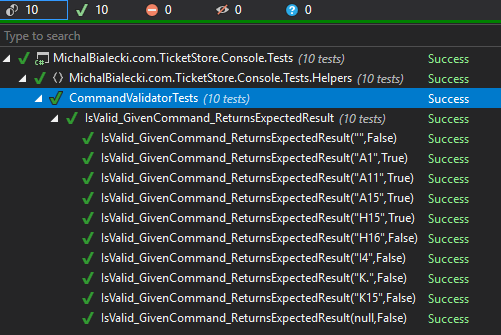
Notice how test results are shown – everything is clear from the first sight. You can immidiately see what method is tested and with what conditions.
If you’re interested what are the best practices to write unit tests, have a look at my article: https://www.michalbialecki.com/2019/01/03/writing-unit-tests-with-nunit-and-nsubstitute/. It will guide you through the whole process and clearly explaining best practices.
All code posted here you can find on my GitHub: https://github.com/mikuam/console-app-net-core