From time to time everyone needs to write simple console application. It’s a great, simple type of project to test something or write a simple tool. However, most of the times it looks… kind of dull.
This is a part of a series of articles about writing a perfect console application in .net core 2. Feel free to read more:
What if it could have an updating percentage value?
Let’s start with something we know. Let’s create a console app, that would show a percentage of the task being done.
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
Console.ReadKey();
ShowSimplePercentage();
Console.ReadKey();
}
static void ShowSimplePercentage()
{
for (int i = 0; i <= 100; i++)
{
Console.Write($"\rProgress: {i}% ");
Thread.Sleep(25);
}
Console.Write("\rDone! ");
}
}
And it produces output like this:
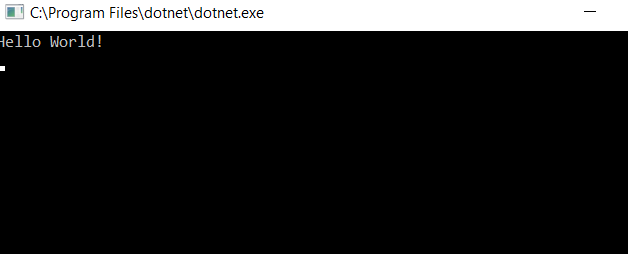
There’s one little trick to it. Notice I used \r character, that moves caret back to the beginning of the line, where next write overrides last one. It’s a simple animation!
Let’s create a spinner
With a bit of searching through Internet and a little of code:
static void ShowSpinner()
{
var counter = 0;
for (int i = 0; i < 50; i++)
{
switch (counter % 4)
{
case 0: Console.Write("/"); break;
case 1: Console.Write("-"); break;
case 2: Console.Write("\\"); break;
case 3: Console.Write("|"); break;
}
Console.SetCursorPosition(Console.CursorLeft - 1, Console.CursorTop);
counter++;
Thread.Sleep(100);
}
}
We will have something like this:
We need to go bigger!
I searched a bit and came across this post on StackOverflow:
https://stackoverflow.com/questions/2685435/cooler-ascii-spinners
Here it is an implementation of an “executive desk toy”.
static void MultiLineAnimation()
{
var counter = 0;
for (int i = 0; i < 30; i++)
{
Console.Clear();
switch (counter % 4)
{
case 0: {
Console.WriteLine("╔════╤╤╤╤════╗");
Console.WriteLine("║ │││ \\ ║");
Console.WriteLine("║ │││ O ║");
Console.WriteLine("║ OOO ║");
break;
};
case 1:
{
Console.WriteLine("╔════╤╤╤╤════╗");
Console.WriteLine("║ ││││ ║");
Console.WriteLine("║ ││││ ║");
Console.WriteLine("║ OOOO ║");
break;
};
case 2:
{
Console.WriteLine("╔════╤╤╤╤════╗");
Console.WriteLine("║ / │││ ║");
Console.WriteLine("║ O │││ ║");
Console.WriteLine("║ OOO ║");
break;
};
case 3:
{
Console.WriteLine("╔════╤╤╤╤════╗");
Console.WriteLine("║ ││││ ║");
Console.WriteLine("║ ││││ ║");
Console.WriteLine("║ OOOO ║");
break;
};
}
counter++;
Thread.Sleep(200);
}
}
And we have multiline ASCII animation:
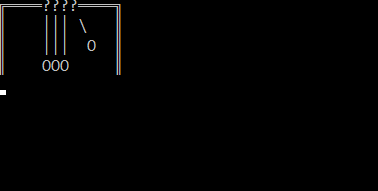
Cool, isn’t it? With your imagination and thousands of Unicode characters possibilities are limitless.
Let’s have some color
Colorful.Console is a fantastic nuget package, that you can use to introduce some color into your console application. With just a few lines of code:
using System.Drawing;
using Console = Colorful.Console;
namespace MichalBialecki.com.ConsoleAppMagic
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("This is a standard info message");
Console.WriteLine("This is an error!", Color.Red);
Console.ReadKey();
}
}
}
We can see something like this:

Colorful.Console overrides original console, so it does have all existing methods, but it extends original object with new possibilities. This is important, because you can start using this console in your old code, but introduce color only where you want it.
So let’s do some animation with color. This is super easy and a lot of fun.
static void ColorfulAnimation()
{
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 30; j++)
{
Console.Clear();
// steam
Console.Write(" . . . . o o o o o o", Color.LightGray);
for (int s = 0; s < j / 2; s++)
{
Console.Write(" o", Color.LightGray);
}
Console.WriteLine();
var margin = "".PadLeft(j);
Console.WriteLine(margin + " _____ o", Color.LightGray);
Console.WriteLine(margin + " ____==== ]OO|_n_n__][.", Color.DeepSkyBlue);
Console.WriteLine(margin + " [________]_|__|________)< ", Color.DeepSkyBlue);
Console.WriteLine(margin + " oo oo 'oo OOOO-| oo\\_", Color.Blue);
Console.WriteLine(" +--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+--+", Color.Silver);
Thread.Sleep(200);
}
}
}
This code produces simple, but effective animation. How would it look in your next console tool? Awesome!
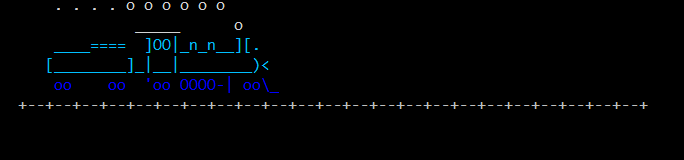
This nuget package offers even more, like colorful text. You can use ASCII fonts to write text. You can download fonts
here – there are plenty.
With a little code:
static void AsciiText()
{
Console.WriteAscii("MichalBialecki.com", Color.FromArgb(131, 184, 214));
Console.WriteLine();
var font = FigletFont.Load("larry3d.flf");
Figlet figlet = new Figlet(font);
Console.WriteLine(figlet.ToAscii("MichalBialecki.com"), Color.FromArgb(67, 144, 198));
}
And downloaded larry3d.flf font file you can see an effect:

Full tutorial is available here:
http://colorfulconsole.com/
All code posted here is available on my github repository:
https://github.com/mikuam/Blog/tree/master/ServiceBusExamples/MichalBialecki.com.ConsoleAppMagic
Enjoy and make exciting and amusing code 🙂
interesting post 😉
I have tried to make you annimation program but cannot succeed. Did you add Using something?
like Using System IO? can you help
Awesome! I did such things a long time ago in DOS… Will be definitely useful 😉
I have a question, is there a way to stop the looping animation? I tried to make a looping animation for the title screen of a game I am making on C# and I can’t figure out how to end the loop to proceed to the next step of the game. Is there any way to do so? Thanks!
Hi Troy,
I think you can add a condition to a for loop. Instead of having only ‘i < 50' you can have 'i < 50 && continueAnimation', where continueAnimation would be a boolean variable. However, for that to work, you would have to have an animation in a different thread. Threading is probably a must-have in game development, but it can be cumbersome at first.