This is a first post about great code review feedback, that I either gave or received. It will always consist of 3 parts: context, review feedback and explanation. You can go ahead and read previous ones here: https://www.michalbialecki.com/2019/06/21/code-reviews/. So
The context
This is a simple ASP.Net application, that is requesting database to get count of elements filtered by one parameter. In this case we need a number of users providing a country code, that is always two character string.
This is how DB schema looks like:
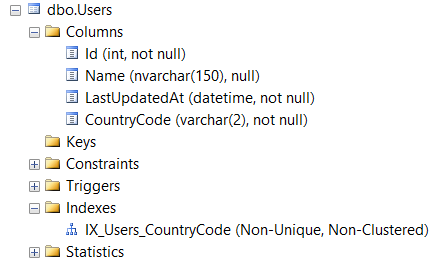
Code in .net app is written with Dapper nuget package, that extends functionalities of IDbCommand and offers entities mapping with considerably good performance. It looks like this:
public async Task<IEnumerable<UserDto>> GetCountByCountryCode(string countryCode) { using (var connection = new SqlConnection(ConnectionString)) { return await connection.QueryAsync<UserDto>( "SELECT count(*) FROM [Users] WHERE CountryCode = @CountryCode", new { CountryCode = countryCode }).ConfigureAwait(false); } }
Looks pretty standard, right? What is wrong here then?
Review feedback
Please convert
Explanation
Notice, that CountryCode in database schema is a varchar(2) and this means that it stores two 1-byte characters. On the contrary
The correct code should look like this:
public async Task<IEnumerable<UserDto>> GetCountByCountryCodeAsAnsi(string countryCode) { using (var connection = new SqlConnection(ConnectionString)) { return await connection.QueryAsync<UserDto>( "SELECT count(*) FROM [Users] WHERE CountryCode = @CountryCode", new { CountryCode = new DbString() { Value = countryCode, IsAnsi = true, Length = 2 } }) .ConfigureAwait(false); } }
If we run SQL Server Profiler and check what requests are we doing, this is what we will get:

As you can see
In order to check how that would impact the performance, I created a SQL table with 1000000(one
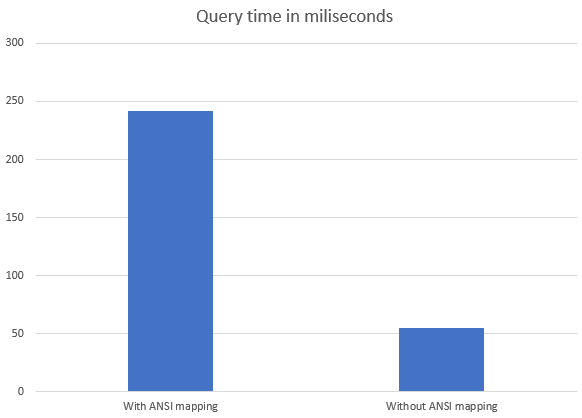
Before review it took 242
All code posted here you can find on my GitHub: https://github.com/mikuam/console-app-net-core